Thursday 6 November 2014
Java Basics Tutorial 3: Switch Statement
The switch statement
The switch statement is a wonderful way to reduce code and make code simpler to read and follow. Let us begin by explaining when a Switch statement would be useful. For example if you had a set of numbers which you needed different results for each. Lets say numbers 1 through 5. Okay there are a couple of ways. An IF statement would do the trick. Ahhh but a SWITCH statement would do the same with less code and be more readable in the future if extending was required to maybe 1 through 10.
This is how they work.
SWITCH (The condition your testing)
{
CASE 1: condition 1 is met
break
CASE 2: condition 2 is met
break;
etc etc....
DEFAULT (a default is set that if no condition is met a result can still be set.)
}
Okay so how does this look in real code? For this example I will do a switch statement on a fictitious game. an imaginary dice throw which certain numbers will let your character move.
switch (dice_roll)
{
case 1:
character = "Move left";
break;
case 2:
character = "Move right";
break;
case 3:
character = "Move forward";
break;
case 4:
character = "Move backwards;
case 5:
character = "Move down";
break;
case 6:
character = "Move up";
break;
default = "Stay still";
}
Well thats all there is to it. Looks clean and simple doesn't it. Really handy thing to know and will serve you well in the future. Just remember a switch statement needs to test a condition. Each case returns a result and you should always have a default just in case no condition is met. Simple!
Java Basics Lesson 2: Operators
OPERATORS
An operator is just a way of testing a conditional statement. To break this down even further an example would be like:
5 < 6.... (the integer 5 is less than the integer 6). The less than sign is the operator!
Okay, pretty simple. We have more operators in Java which make coding a conditional statements very simple.
Other conditionals in Java can test other things. Here are a few:
< less than
> greater than
= equal to
<= less than and equal to
>= greater than and equal to
!= not equal to
++ increment integer by one
-- decrement integer by one
&& AND
|| OR
We generally use operators in programming to test a condition. If a person is a girl or a boy, if your pet at home is a cat or a dog... all of these things can be tested in programming through conditional statements.
How we do this is: (example show how to test what stage of a boys life he is in (String stageofperson))
if (boy_age > 12 && < 18) //if the boy's age is greater than 12 AND less than 18 he is a teenager
{
stageofperson = "Teenager";
}
elseif (boy_age >= 18 && < 70) //if the boy's age is greater than or equal to 18 but less than 70 = adult.
{
stageofperson = "Adult"
}
elseif (boy_age < 12)
{
stateofperson = "Child"
}
else
{
stateofperson = "Pensioner"
}
WOW okay thats looks long but actually its not. We are checking through an if statement to see what stage of the person of life is in. Child if he is less than 12. 13 and above to 18 makes him a teeenager and 18 and over but less than 70 makes him an adult. If none of those match he must be older than 70 which makes him a pensioner. Seems like a long convoluted way but it actually makes for simple and easy programming and simple to explain here. In real life coding practice we would use a SWITCH statement for the above code. This I will blog next but for the purpose of understanding conditional operators this serves it purpose.
Another example would be this in a WHILE loop. We will to WHILE loops soon...
while (name != "Jenny")
{
//increment the student number
student++;
}
Well hope this has helped. Operators are used in every single program you will make. You will know them soon enough. Do not be daunted by anything here.... it will all come together quickly and soon.
Saturday 1 November 2014
Java Basics Lesson 1 - Variables and Data Types
Variables and Literals
Variables are dynamic and can be changed. Variables basically hold data or information. Think of them as slots in a memory bank. Variables can be assigned values easily and they hold that data until they are changed or discarded as redundant.
A literal is a fixed type of data (the types of data that can be stored in a variable). There are four types of literals. These are:
- Booleans (True or false)
- Characters and Strings (character is a single letter, Strings consist of usually more than one character)
- Numbers (Java supports integer, floating point, byte literals)
- Nulls (The Null literal is literally what it means...nothing)
Examples of all above:
boolean hairywoman = true;
char initial = p;
String surname = "rabbids";
int integer = 3;
float floating = 3.1467788557757785464434 ; (floats can hold 32 bits of data)
double mega_floating = 3.4e+038; (doubles can contain up to 64 bits of data)
byte byte_literal = 2; (nobody really uses bytes anymore as integers do the same job)
When we are coding we will often need to hold information that a method or part of the program will require to use later. Variables do this efficiently. You can use them in multiple ways to achieve a goal for example.....
int a = 5;
int b = 3;
int result = a + b;
the result variable now holds an integer 8.... clever stuff!
you could then use a boolean in a loop (we will get to loops later)
if (result == 8 )
{
boolean answer = true;
}
else
{
boolean answer = false;
}
Thats all there is to it folks!
Thursday 23 October 2014
More German advert humour
Another classic advert from Germany. This one is a fairly old advert but just has always remained in my mind as a classic. Enjoy by clicking the link below or watching it on the youtube insert in the page.
Real leaked court document makes for funny reading.
Somethings you see on the internet and you really and truly are astonished with the stupidity.... this image below is a real document of a conversation in a courtroom between the Judge and a witness whom is a Doctor.
Read the image and try not to laugh as this friggin happened...... Pleased the Doctor saw the funny side, the judge is probably still thinking!
Wednesday 22 October 2014
Saturday 18 October 2014
Choosing an IDE for my Java Application development....
I occasionally code for android and use the Android Studio platform. This platform has come a long way since it's beginning only a year ago. The Android Studio has been coded and ported from the IntelliJ IDEA (www.jetbrains.com) community. I really do like this IDE for Android so I installed it on my Linux laptop without hesitation. Unfortunately it seems for simple Java Swing development it is overly complicated and took a long time to set up to even begin coding. They also have a unique way and structure in dealing with Java projects that you create. It was not long before I was looking for a quicker more straight forward solution as I am not a heavy developer. I may return to this IDE at a later date.
Everyone knows of Eclipse, Eclipse has been the IDE of choice for multiple programming languages, it is heavily supported and there are a bunch of docs and community aid online. I have used it before and it's known for having a couple of weird bugs here and there which can be frustrating if you do not know all of them. Thus, you can be looking at fault in your code for days to finally find out that the null pointer exception was a compiler error (might be a poor example but think this has happened to me on several occasions). I also do not like the feel of Eclipse visually or intricate design layout.. So Eclipse is a bit like Marmite. You love it or hate scenario. I am impressed with it...but I don't enjoy to use it.
NetBeans is what I have ended up with. There are others to choose from but it just seemed logical to myself to use a dedicated Java Platform IDE for developing a Java Swing Applications. I have installed it as I did with the other two and found I was rooted into coding my new app instantly. It looks like a basic IDE and it seems to have all the features of both mentioned above for what I am doing, I also managed to get started, designing and coding my app within minutes of installation. I was at least one hour on each other platform before I even started to look at coding. No imports or plugins needed just a raw coding platform with a GUI designer. Simple. I downloaded the latest version as the one Ubuntu have in their repositories is ancient. Years old.... sort it out Ubuntu. So hats off to my new IDE of choice NETBEANS! You made things easy and I am enjoying coding together my app. Wish I only had more of a clue about Java. Referencing has become a 3 minute habit. Brain is fried.
FILE KILLER Alpha .007 in development.

Sunday 12 October 2014
Drive Club... worth the wait?

What about the Cars and the Tracks? Pretty important in a racing game I would say. Well this is a
mixed bag. The Cars are perfect replicas. You could not tell any details which defect from the real thing. There is a good selection of cars but not hundreds. A few hand picked great cars worth driving. They have modeled them so well I can see why they did not go crazy building lots of cars. To build one of these must have taken forever. So hats off to the developers for this. The tracks are another story. No real world maps are included. None. Not one. This for me was a massive let down. A huge hole in a racing game. They have made up their own tracks. None of which flow well or give you that technique and feedback making you feel the need to master the road ahead. The tracks are mainly just sections of road in what they consider exotic locations, Scotland, India, Canada etc.. In those Countries there are a couple racetracks rather than roads. The tracks again are fictitious and lacking. They have no feel of a real racetrack. Poorly designed and I can't see how they will create great racing either. I have driven on them all and none have grabbed me as " a track I gotta improve on". Okay, so Cars look good and the tracks suck for a dedicated racer.... what's the actual physics like...?
Damage on the cars is present which is a welcomed addition to these types of games. I know that manufacturers generally do not like Game Studios adding real world damage physics as they want their car represented in the best possible light. Well Drive Club have managed to somehow get over that with the manufacturers and included a limited damage system. Although I say damage is included we are talking purely aesthetic. You can drive as fast and as hard as you like into any object without any real threat of anything except for loosing time. Once again very disappointing. Not every person will agree with me here...many find car damage very frustrating which I understand but at least turn damage off. At least have an option. Another let down which makes this game a stupid arcader dressed up to try and be something it will never be.
I am also disappointed with their method of starting a race. No Qualifying, just a random place on the grid which is never pole position. No lights, not even a countdown, just "READY" and when that disappears, which happens way to soon, the race has begun. Their is no feeling of lining up on a grid, the need for a perfect start, any tactic to be thought about for heading into turn 1... its all just go and lets smash everyone out of the way as I dont even bother to brake for turn one...who cares? no damage, no costly effects, no thrill, no need to race. The more I write the more I hate this game. I am actually getting more and more disappointed as I write.
Well okay it's just a really pretty arcader, but isn't this all about the online elements of the game? Oh
my god...where does one start. The Developers have apologised....... their game doesn't work online yet. Yup.. thats the short of it. Its an online racer which is not working. If you have seen the news they are struggling to maintain their servers and they have all been overwhelmed. Okay... be slightly sympathetic, all games have a bump here and there on release. Battlefield 4 was the biggest bump I ever had hit before. Drive Club takes the biscuit. They have actually had to cancel some parts of the online play until they can fix it. Oh dear, the problem is you can't even get online. The servers are down all the time. If you do manage to get online... the online racing is no better than any other game. They have taken a new angle to try and build online clans and teams to drive together in. I just cant see how this will hold any value if the game itself is not playable to any standard of a sim. Arcade bashing and crashing offers no skill reward and no reason to be in a team or even try to work together. Their is zero realism so why bother.

Will definitely be interested in "The Crew"... will review that when I get the chance to play it in on release day. Sounds like an arcader which offers a lot more than this!
GRAPHICS 9 / 10
GAMEPLAY 3/10
ONLINE 3/10 (if you can get on)
PHYSICS 3/10
TRACKS 3/10
OVERALL 4/10
Tuesday 7 October 2014
Twitch's popularity grows but what is it?
Twitch is growing in popularity fast. So fast in fact people are now actually starting to earn money by playing video games and streaming continuously their gameplay. They respond to comments and have a screen capture of themselves making a unique advertising possibility appear. Some gamers are even sponsored and you can sponsor them to play which will ensure that their channel of game streaming will continue. We have all seen the adverts in YouTube, well think of this as a dedicated gaming advertising platform.

So other than looking for tips and advice, tactics or cheats what else is capturing audiences in such great numbers to watch video gameplay? There is an interaction with the gameplayer (host) you can chat through voice or simply type text into a message window which the user can hear or read and respond to you. I have often also watched games which I thought i wouldn't buy or play and actually found that I like the game. So I find myself looking at Twitch to see gameplay of a game to confirm my decision about which games I purchase. Long gone are the days of the demo.... so this really is a neat alternative.
One of the greatest and probably least recognized feature of Twitch is the fact that you can actually see games before they come out. This is amazing. You can actually see gameplay of games which have not even gone to gold (been printed on CD). Games like Elite Dangerous and Drive Club are often viewed as developers and testers air their game time on occasion. This is a real sneak peak into the actual progress and gameplay and I feel gives more perspective of the finished product than a E3 display.
So to sum it up Twitch, although an unlikely business model has proved with great success that this unique platform is in demand. For a multitude of reasons seasoned game players all over the world are finding they are tuning into Twitch more and more. Hints, tips, tactics and just for the hell of seeing someone totally make a mess of things in GTAV will keep the masses entertained more than they realized. I really did not expect to enjoy watching other people play.... I was wrong!
Sunday 5 October 2014
Google talks with CyanogenMod....
Just a quick news flash... Recently heard that Google had been talking to Cyanogenmod with the rumor that discussions were put forward to buy out the most popular Android ROM maker. Now the only reason we can tell they would buy it is solely for the purpose of closing down and locking the doors of one of the greatest branches of Android's open source.
It is obviously not that straight forward... I'm sure the Cyangenmod team would have been incorporated into the Google team as their is no denying just how brilliant the developers at Cyanogenmod are. Google would only benefit from their coding practice and ideas.

My thoughts are the Cyanogenmod team have stood strong in the face of corporate domination and have not sold out as they knew it would mean closing the doors of that wonderfully successful team as we all know it.
We know Google are just about to launch a new version of their Android system which does not include Dalvik runtime but a new ART runtime environment which is far more energy efficient and process and RAM friendly. With this new system Google are trying to lock down the current fragmentation of the award winning and popular OS. They feel they need more control of their system. Even although Android is based on Linux and the very idea of Linux is many hands make light work and further improvements are executed by the users.... this may not be so beneficial to the Android OS.
We shall have to wait and see if the Cyanogenmod team can hold out or if Google's strong buying power will see that our favourite open source ROM will come to an end. Either way...Android will improve and the Cyangoenmod team can only be proud of everything they have done.
Thursday 2 October 2014
Linux - Bash's forgotten super tips!
![]() |
Bash in Linux running the htop command |
Before responding in the comments about 50 million other tips I forgot, I thought the one's I have picked are useful to a new user and which are commonly never remembered but can make life super easy in the land of BASH.
(ctrl R) press this whenever you want to find that command you typed in but cant remember. It is Bashes reverse search of previously typed commands. Just type in the start of a command and up comes the rest.
(ctrl U) just typed a whole line and its so long you think you make break either the delete key or your finger to clear it... ctrl-u will instantly blitz the line as it it never existed.
(ctrl W) damn number 2 s handy but I only messed up the end of the line.... ctrl-w will delete the last word of your command line entry.
(pstree -p) simply outputs in Bash your process tree from the cpu. Handy!
(lynx www.yoursite.com) Download lynx right now. This is a full blown web browser in Bash. Super cool if you only have a terminal to work with. Lots of power and just a neat different way to view the web. Get it: sudo apt-get install lynx
(lsb_release -a) Instantly find out your distro version and codename. Useful to know if your on the help forums.
(htop) Useful System Output where you can kill,pause,or modify processes and see cpu usage and memory usage. In fact a ton of info is in htop. Get it: sudo apt-get install htop
(ctrl U) just typed a whole line and its so long you think you make break either the delete key or your finger to clear it... ctrl-u will instantly blitz the line as it it never existed.
(ctrl W) damn number 2 s handy but I only messed up the end of the line.... ctrl-w will delete the last word of your command line entry.
(pstree -p) simply outputs in Bash your process tree from the cpu. Handy!
(lynx www.yoursite.com) Download lynx right now. This is a full blown web browser in Bash. Super cool if you only have a terminal to work with. Lots of power and just a neat different way to view the web. Get it: sudo apt-get install lynx
(lsb_release -a) Instantly find out your distro version and codename. Useful to know if your on the help forums.
(htop) Useful System Output where you can kill,pause,or modify processes and see cpu usage and memory usage. In fact a ton of info is in htop. Get it: sudo apt-get install htop
(mtr www.yoursite.com) a simple traceroute to help you figure out where your network is hanging. Very handy.
(whatis command) Not sure what something does? then type into Bash whatis then the program. It will quickly tell you what that command is for. e.g whatis tar tar prints this (1)- The GNU version of the tar archiving utility
And finally (wc filename) Word Count. Take any document and type in wc -option(*) filename and you can get instantly what your after... here are the (*)options....
-c print the byte counts
-m print character counts
-l print new line counts
-L print the longest line length
-w print how many words
e.g wc -w bookmarks.html (answer returned 3500 words)
You can also add multiple files.... e.g
wc -w bookmarks.html pringles.txt suicidenote2.doc and it will give you the results for each.
Well thats my super handy top forgettable Bash tips for now. All super useful and easily forgotten to the newcomer or even some bearded linux historians occasionally forget ;)
Tuesday 30 September 2014
A Google account comes with a ton of FREE services....
Regardless this is not what this blog is about today. What I am wanting to blog are some of the Google services I use on a daily basis. Later I hope to do some in further depth tutorials regarding use of some of these services e.g Gmail and Drive etc.....
Firstly I would like to list the services available which I use a lot and I would also like to explain these are totally free. 100% without cost to you and provide amazing functionality and productivity if you can be bothered to utilize them. The list goes like this:
- Gmail
- Drive
- Google Plus
- Google Photos
- Google Search
- Calendar
- Maps
- Sites
- Keep
- Blogger
- Shopping
- YouTube
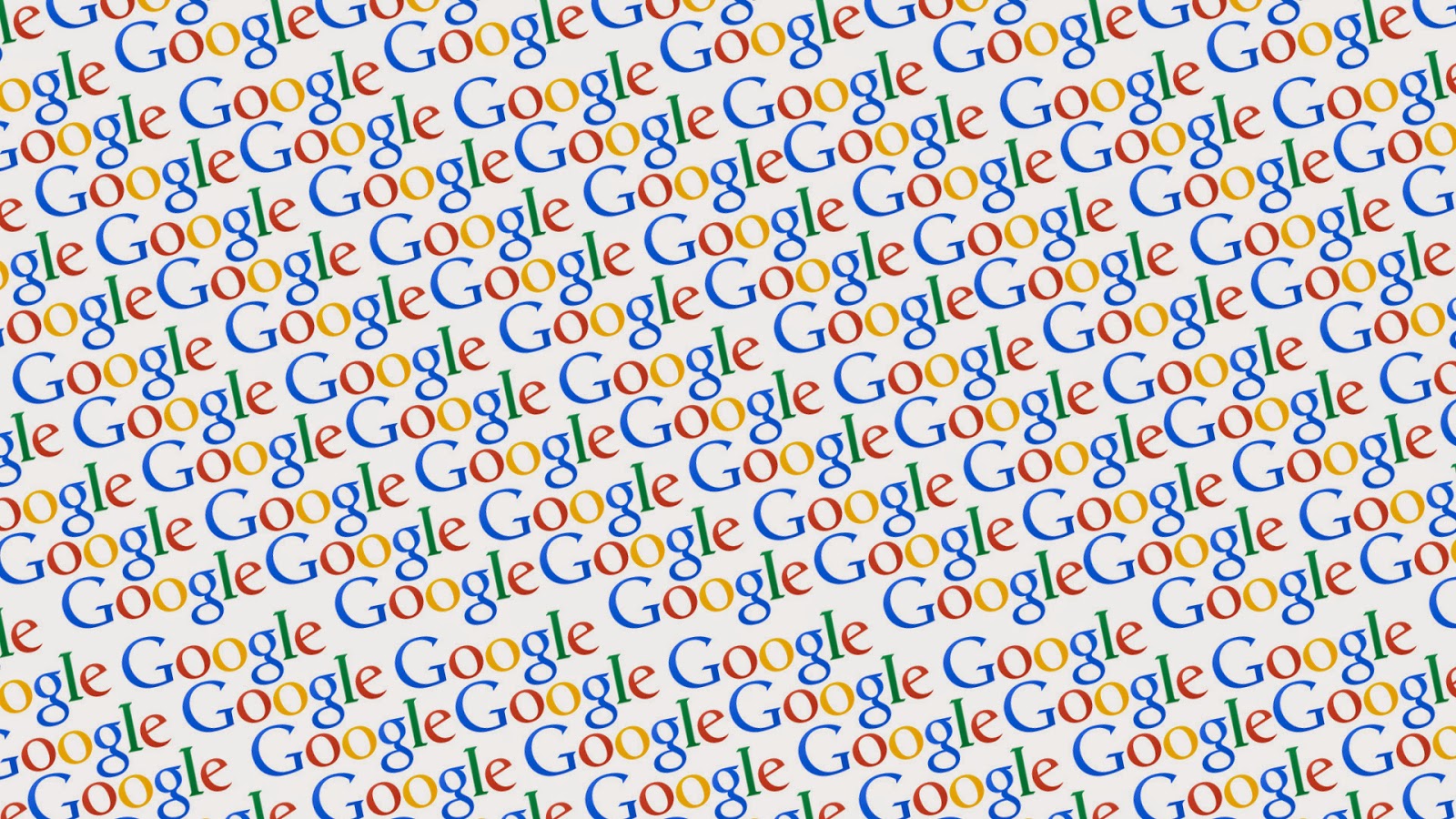
Going through the list now iI will quickly describe each:
Gmail
Web Based email. Log in from anywhere in the world and have the convenience of having your email and being able to send emails.
Drive
A Cloud Service for storing files which can be then downloaded and used anywhere on any computer. Also files can be shared with colleagues or friends if you desire. Strongly integrated now with document support. You can now scan in documents using the camera on your phone, edit documents in many formats, use spreadsheets and even create presentations. Google really have got the upper hand on any cloud service available on the current market.
Google Plus
Google's version of Facebook. a community driven sharing facility with many more benefits than its' competitors. Follow people of interest, follow your friends, add them to your circles and keep updated with anything that interests you. Far more focused on information you want than the competitors shoving rubbish down your throat all day. A really under appreciated service. Unfortunately Facebook wins with dedicated users but really Google Plus is far superior, people need a little patience to understand it that's all.
Google Photos
Photos is exactly what it says. A wonderful place which integrates with other Googles services like Drive and Google Plus. Photos will store all your photos which you can put into albums. If you have an Android phone you can select auto backup and every pic you take will be safely stored in Photos so if the unfortunate happens (phone stolen or dropped in the drink) then you will have not lost anything but the hardware.
Google Photos also has a strong editing suite where you can enhance, rotate and colour adapt your pictures with great success. Another strong package from Google without a doubt.
Google Search
Quite simply the best search engine on the market. It delivers accurate and topical search results in an instant and even helps finish looking for something before you have even typed it in. There really is no competitor.
Calendar
All my events go in my Calender and my Calender is shared with my wife. All events appear on both our phones and tablets. The Google Calendar service is so useful. Sharing Calendars is just one of the many features. Organize your days, even receive emails or alarms for upcoming events and build task list and coordinate events with others. Its a daily requirement for me and without it I would have missed my own birth!
Maps
More accurate than any other map program open to the public. It's definitely more superior than my Honda Sat Nav which on several occasions has tried to kill me! Google maps is more than just a map, it offers an entire in depth yellow/white pages of local businesses, perfect directions with multiple modes of transport, wonderfully detailed satellite views and a street view which is amazing. I live on this app. It saves me more time and removes more anxiety than I can imagine. Have no idea how I lived for so long without the service.
Sites
Google lets you build your own website for free. Handy if your a small business owner. It's not the most in depth service and it does not provide lots of functionality but it will give you a web presence. Not only that they have some simple templates to help you get started.
Keep
The ultimate in note keeping. Think of it as digital post-it notes with a lot more functionality. Add voice messages , pictures, lists or just plain texts. I use this regularly on my phone and laptop. Its great for making quick info available everywhere. Any note I make appears on all my devices from phones to laptops. Super handy for making shopping lists. I go shopping and by the time I arrive at the store my wonderful wife at home has added everything we need on the list. I just go around with my phone and check each one off as I go. My wife then knows where I have got to on the shopping list as the 'ticked off' items disappear from the list, she can also add anything she forgot if she catches me before I leave the store of course ;)
Blogger
Right now you are reading this on Blogger. Blogger is only useful to me as I run a blog. If you have an interest in running a blog, then Blogger is free, comes with pre in built templates and the new Blogger site can also let you customize your blog. Mine is customized to my liking. Hopefully you like it too.
Shopper
Google Shopper is found in the tabs after you have made a search. Or you can search directly for a product on the Shopper tab. This will return results of the product your searching for and the prices. Often I find products closer to me in stores than where I was originally looking to purchase the product from. Convenient and very easy to use as a price comparison between major stores. You can save yourself a small fortune just by using this service.
YouTube
We all have used YouTube at some point in our lives. It has probably been the largest revolution in technology and the internet in the last 15 years. YouTube has inspired young film-makers, crazy and funny videos, music, advertisers, viral videos and so much more. People are desperate to share and a lifetime could be wasted looking at YouTube videos. Really an amazing service. Upload a video, watch thousands of videos and comment on other videos. Subscribe to your favourite video posts. I use YouTube everyday. I use it for everything from making me laugh to viewing a tutorial on programming or even diagnosing issues I have with my car...... Its an amazing service....think I said that!
I do use other services not mentioned above but these are my daily uses. All free and all come with a Google Account. An amazing company with a real touch for inspiring a technological future and I only wished that more companies developed their services like Google do for us.
Monday 29 September 2014
Bash in Linux Bugz (SHELLSHOCK)
![]() |
Bash Running in Linux |
"In a nutshell, the Shellshock Bash bug is easy to exploit and yet still have severe consequences".
Bash is often used as the system shell in many distributions of Linux and Macs. IF an application calls a Bash command from the internet (Http) or through a common gateway interface (CGI) which will allow user to enter data, that system is open for attack. The vulnerability will affect thousands of applications across the board. Andy Ellis, the Chief Security Officer of Akamai Technologies, wrote: "This vulnerability may affect many applications that evaluate user input, and call other applications via a shell."
So have updates come out. In most cases your distribution should have updated bash packages for download. Certainly updates have happened at my end using Linux Mint and Fedora were quick to act too. The most shocking part of all this news is that it seems all companies have responded treating this "critical" bug as something to be addressed immediately, except Apple have done nothing. No patch has been released for Mac users. Also Apple have not said anything about releasing a patch immediately.
Crikey? Well how the hell do I know if it affects me. Well if you have not updated your computer in the last week....then it affects you. To be sure though you can copy and paste this into your Bash terminal to see if the vulnerability exists....
Are your computers vulnerable?
Open a terminal window and enter the following command at the $ prompt:
1
|
env X="() { :;} ; echo ALERT" /bin/sh -c "echo ASSCOVERED"
|
If your answer returns this:
ASSCOVERED
Then your good to go. You have been patched in an update.
Sunday 28 September 2014
Kazam - The ultimate screen recorder for Linux
![]() |
Kazam Screenshot taken of my laptop (Linux Mint) |
A very quick review and tutorial for those interested in screen recording within Linux. Kazam is a great little program which runs in the background recording a video of your desktop and all that you are doing. This is a great way to do tutorials on software usage or live recordings of games being played which is known as "Screen Casting". Many of my blog posts will include a Screen Cast as it is a great tool to physically see whatever I am discussing removing much of ambiguities and misinterpretations.
Included is a YouTube video of Kazam recording my desktop and in the audio I shall give you a quick tutorial in how to use it and the programs features. So how do you get it? Simplest way to download Kazam is through the Ubuntu repositories.
Open a terminal and type:
- sudo apt-get update; sudo apt-get install kazam; sudo apt-get autoclean;
If you're not Ubuntu based you can get Kazam from https://launchpad.net/kazam here you can get a tar.gz copy to build from source.
So what are the features of Kazam?
- You are able to record the whole screen output, a specific application box, or even create a capture are by highlighting a section of your screen that you wish to be captured.
- Several different video formats to be output too. I mainly stick with the h.264 codec and record my files into mp4. "YouTube friendly".
- You can choose the frame rate of your capture so you can control the video smoothness or if you are on an older computer that it can keep up with the recording.
Other features also include CPU parameters to once again alleviate CPU burden. File output stowage location changes. Countdown timer on screen to give you time to prepare before screen casting begins. Small task bar icon so recording can be stopped, paused or continued easily.
There are other Linux screen caster's but Kazam fits the bill for my requirements.
I also find it is a regularly maintained package and development is still in progress which gives me hope that this wonderful app will continue long into the future.
Friday 26 September 2014
DOD LS 300W Dash Cam Review
DOD LS300W Car Dashboard Camera Full HD 1080p Advanced Super Night Vision 2.7 Inch LCD 140 Degree Lens G-sensor Motion Detection
Unfortunately driving in the UK is not improving. More cars on the road and less enforcement picking up on minor highway code breaches. Thus, we have become a nation of lazy drivers. I have been involved in an accident many years ago where if I had on of these cameras I would have not had to defend my claim that the other driver crashed into me. In fact that the other driver ran me off the road after crashing into me and my car was a write off. Due to the fact there were not any witnesses and it happened in a single lane county lane meant that the insurance companies settled it as a 50/50 fault claim. This obviously made my blood boil.
So I bought one and have fitted it. Here is my review.....
The camera performs well. I looked at many and checked out a fair few dash cam reviews on you tube before deciding that this was the one I would try. I saw many for around £40 but after reading customer reviews on these products most complained most complained that the picture quality was too poor to see a number plate or did not perform at all at night and the camera had lines through the picture when in bright sunlight. There were many other cameras which were higher on price too. £300 some? I was not willing to part with that much cash. So I did a compromise. £96.99 is what I paid. I purchased it from Amazon.co.uk.
The dash-cam arrived within a couple of days and I had it fitted in my car with all the wiring hidden within 2 hours. Could easily be done in an hour just a few cups of tea's and neighbours chit chat got in the way. I bought a 16Gb micro SD Card and inserted it in the side. The manual for the camera was non-existent but thankfully it is so self explanatory no manual is really needed. The camera's menu system is very easy to understand. The camera goes into a loop and just records continuously. If you want to lock a file which then can not be overwritten, this is possible. I turned off the G impact sensor on mine and also disabled the light that is on it. Neither are really of any use to me. The camera itself does have an internal battery but I run it of my cigarette 12 volt socket. The inbuilt battery does not last long anyway. I also turned off the microphone as I don't need all my conversations between my wife and I recorded or worse my screaming daughters!
The image quality is brilliant for the money. The camera adjust to light instantly and does not distort like many of the other camera's I saw reviewed on YouTube. When driving in the night, the camera picks up the road extremely clearly just from your headlights. During the day in all weather the video is extremely clear. My overall review is this will be an invaluable tool if ever required by an insurance company. Personally, I think insurance companies should offer huge discounts to drivers that use a quality or approved dash cam when driving. If we all had these... I feel people would drive with more attention and be more aware that if they are doing something stupid....there is no lying or distorting any truth to the insurance company or police.
Strongly recommend one of these little cameras to anyone. Below is a small clip of a drive I did in the morning ;) Best watched in full HD 1080.
SPECS:
- 1.*140 degree wide angle lens *FULL HD 1080p *2.7" 16:9 Widescreen Display*Six-Element Sharp Lens - Crystal-Clear Image
- 2.*Advanced WDR Technology - Capture Light in Control*F1.6 Big Aperture - Super Low Light Performance*Appearance Patent
- 3.*Loop recording, Time and date Stamp*Motion detection recording function *G-sensor impact data protection *Stop voice recording function
- 4. *Support to 32GB Micro SD card*SOS file locking function *Start and record automatically function*Start and record automatically function
- 5.*Package included: *1 x DOD LS300W Car Dvr Recorder *1 x User manual *1 x Bracket *1 x Vehicle Power Charger *1 x USB Cable *1 x HDMI Cable
- Product Dimensions 11.4 x 4.8 x 3.7 cms 73 grams
- Boxed Product Weight 499 grams
Wednesday 24 September 2014
Honda Civic 2009 personal review
How come I ended up choosing, it was a choice, a Honda Civic? Straight away I can answer.... Reliability. Reliability goes a long way after what I just went through with the Audi money pit. Honda have quietly sat on the top step of the podium for most reliable manufacturer of cars for 8 years. Who knew.... We all knew they were good but 8 years in a row as most reliable car is really something.
Was it just the reliability I was after? Of course not. I love driving, I am one of the few who really takes pleasure in driving. I am also a new father and so practicality had to factor in the decision too. Honda are known for their practicality too. I know this as I lived in Japan for many years and practical was the name of their game. I then started to look at parts....as I know my luck....I could possibly buy the one and only Honda which defies their 8 year record. So I decided if that's the make of car I will buy it from the dealer directly...one year warranty on approved Honda's.
What about other cars that took my fancy. Yep, sure there was a few. I did not want anything from the VAG team so Golfs, Skoda, VW were out. That left me with Volvo V40 or v60. Neither appealed to me and supposedly they had their own issues with sensors. I looked at BMW 3 series estate's. Nice car but servicing and parts were astronomical. I looked also at Peugeot and Renault but really they were never on my want list.
I took a trip down to my local Honda dealership after looking at some car reviews online. I still was not sold and needed to see the car for myself. I really though it was gong to be too tight on space. I made sure I took my stupidly over sized, all whistles and all bells, dancing pram. Biggest hindrance to any car. I was approached so I asked if I could see the Honda Civic. At the time they had just received the new Honda Tourer. Got to say.... expensive but looks good. £30k though puts it in the high end. Anyway I only had about £9k after my last car experience. Back to the point, I took the pram and looked at the car... no way was it going to fit in a Civic. I opened the boot and the pram slid in with room to spare. Plenty of room to spare. My A6 had a big boot. Not as big as this. The car was tiny though? How does that work? Not only that the guy said you don't have to put it in the boot you know.. I didn't know what he meant, I said I don't want to lie it on the back seats. Don't have to. He showed me how the car seats not only lie completely flush down if you want to extend the boot, but also how the seats can be moved up in the middle... basically removing the rear seat of the car leaving enough middle space you can put what you want anywhere. I was amazed. They really had thought about this car.
outdated in its maps though and also isn't the best system I have used. Google's Maps is the best to date. The voice control in the car either works or doesn't for your voice. I still find it a bit hit and miss. Their are a ton of commands to learn and any slight deviation from the exact command and it poops out straight away and start talking German or Spanish back at you. Concerning! The phone blutooth system hooked up well with my Android phone although it didn't download my contacts like other I have used. Room for improvement but functional.
- Looks - 7/10
- Drive - 7/10
- Practicality - 9/10
- Economy - 8/10
- Comfort - 7/10
- Gadgets - 9/10
- Value - 9/10
Tuesday 23 September 2014
Android Apps which I use daily and why?
My choice of apps on the Samsung Galaxy Note 2
In this I will show you screen shots directly off my phone (Samsung Galaxy Note 2) which will display which applications I have installed. I will explain why I chose the applications I have and maybe one or two could inspire you to use them too.....
Screen shot 1
- Adobe Reader - Why use anything else to view pdf files. Its an Adobe standard!
- Airdroid - Conveniently hook up to your phone through a wireless connection from your PC. Send and receive files between the two mediums and easily send texts from your laptop and so much more. This app is a must for me.
- Amazon - It is just a great way to compare prices and easy to shop from. Not always the best deal but their services are second to none.
- Android IRC for SlimRoms - Non essential irc chat program for people who use SlimRoms. Me. Its on all my phones
- Blogger - Well I write a blog so this is the official app to do this from my phone. It is not great but it's something.
- Bluetooth File Transfer - Just in case I have to send something to an old old phone or my mom's IPhone.
- Browser - Do not use it. Use Chrome
- Calculator - Everyone uses one at some point
- Calendar - Synced with Google keeps me from missing nearly everything.
- Camera - Takes good snaps but I prefer Google Camera which is the next Icon
- Changelog Droid - See what updates happened and actually what changed with the app.
- Chrome - Definitely my browser of choice. Plenty of pro coders at Google only making it better too.
- Clinometer - Pretty much a bubble level tool which is very handy around the house.
- Clock - Time / World Time / Countdown Timer / Alarms
- Dev Tools - When I need in depth info about my phone for development purposes
- Device Manager - A tool to remotely wipe your phone, track your phone and call your phone if you have misplaced it or it was stolen.
- Docs - Google Docs are a fantastic creation. Carry your documents and make documents on the fly.
- DSP Manager - I don't really use this.
- eBay - Who doesnt like to bid. Always have used the official app even although alternatives are available.
- Elite Companion - Is for the upcoming game Elite Dangerous. If you dont know what I am talking about read my Beta Test review here on my blog http://unleashedcode.blogspot.co.uk/2014/09/elite-dangerous-not-ready-but-obviously.html
- Email - I no longer use any email apps other than gmail.
- ES File Explorer - Simply the most powerful and usable file explorer fro Android.
- Facebook - The facebook app is the genuine app. I would like an alternative but unfortunately every alternative I have tried fell short. I used to use Klyph but it is no longer supported.
- Feedly - This is what I used ever since Greader went down the pan. Best news reader out there and with a great interface.
- Flib - A simple conversion program but is asthetically pleasing even if it is a little confusing
- Flixster - Great app to see what films are good and what films are flops.... Can save you 2 hours at a time ;)
- Gallery - The standard gallery app for local (phone based) photos.
- GMail- My only mail system. Dont bother with any others
- Google+ - Considerably a better platform and design to Facebook for social networking. Even although Facebook ha a greater audience.
- Gumtree - Basically free ads on your phone. See whats local and pick up a bargain.
- Hangouts - A brilliant app by google. I use this religiously and speak to people in all parts of th world on video chat for free on my phone and laptop
- IGN - Good to keep up to date with the latest in games. Not always the best for reviews though
- Keep - Google keep is my favourite of all apps at the moment. I have it on my laptop and on my phone. Any shopping list or quick note is added and I can see it on either platform.
- Maps - You cant knock Google... their maps are simply far superior to anyone elses. The information included in this one app is astonishing. Its incredible.
- Messaging - Send a text
- Messanger - Facebooks messenger service. Compulsory by Facebook and not many people are happy about it having to be a seperate app on their phone. I am one of them. Shocking!
- News and Weather - Goggle's news and weather app. Of some use sometimes.
- Nova Settings (Nova Launcher) - My Launcher screen and standard with SlimRoms. Really a very good experience all round.
- PayPal - Check my PayPal account and pay for things from the convenience of my phone.
- Photos - Google photos shows local phone based photos and pictures in your Drive and Google + albums. great app but could be improved.
- PlayStore - Goggle play store for downloading apps, music, books and more
- PlayStation - This is the official PlayStation app which links to your PS4 and you can view your accounts and messages
- reddit - I occasionally use reddit as it is a good source for finding articles or pictures of the unusual
- Sheets - Google Sheets is the spreadsheet of choice on a mobile. Completely compatible with most formats and saves to your Drive. Handy, handy tool.
- Sky+ - This is so I can see what's on TV. Also lets me remotely record programs that I do not want to miss. You need a Sky Plus account to use this app.
- TapaTalk - One of the best apps if you regularly read forums. Forums can be slightly hard to read on small screens and this app eliminated that problem. Great app.
- Torch - Saved me a few trips in the night. Highly reccomended.
- Tumblr - A really good blog/photo site. You can search for almost anything on Tumblr and get many hits back with content. I use this app a lot.
- Wifi Analyser - This is one of my most helpful tools. Making sure I am not on the same channel as others and making sure my wifi remains problem free around the house.
- YouTube - We all know and love YouTube....except for the adverts! Still we all use it daily.
* Please note you may have noticed that some apps were not included but are on the screenshot. This is because I didn't feel those apps needed any explanation as they are standard on almost every Android phone.
** Please also note since the writing of this I lost my patience with the Facebook app with its decision to force you to download another messenger app which is extremely invasive on your screen. Although it is not perfect it is the best I can find for now is FriendCaster. It is the closest we will get to the all out app in in many ways better. Just misses a couple of features like editing after a post has been published.
** Please also note since the writing of this I lost my patience with the Facebook app with its decision to force you to download another messenger app which is extremely invasive on your screen. Although it is not perfect it is the best I can find for now is FriendCaster. It is the closest we will get to the all out app in in many ways better. Just misses a couple of features like editing after a post has been published.
Subscribe to:
Posts (Atom)